Let’s take a look at how we can use code to represent algebraic expressions!
Go to this website:
https://cscircles.cemc.uwaterloo.ca/console/
1 – Type the following into the Console:
for termNumber in range (1,10):
termValue = 2 * termNumber
print (termNumber, ‘\t’, termValue)
Keep in mind that in coding, words are case sensitive, symbols must be exact, and indentation is important. Don’t worry – you’ll get used to this if coding is new to you.
Here’s what it looks like when you’ve typed the code into the Console:
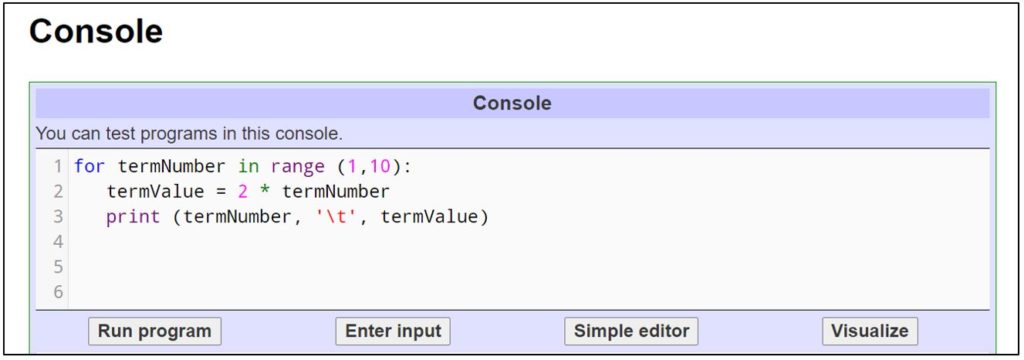
2 – Click on Run program.
Your output should look like this:
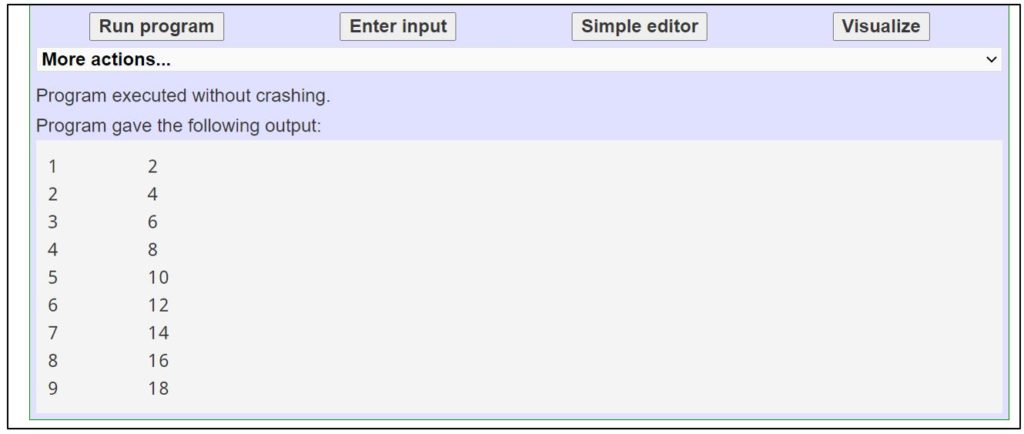
Often, when we are coding, we will get an error message. This error message helps us to determine which line of code contains an error. Typically, people might leave out a colon or spell a variable name wrong. Double-check that you’ve typed everything correctly and click on Run program again.
Notice the output contains a table of values.
The first column contains the term number and the second column contains the term value.
The asterisk (*) means to multiply in coding.
The expression shown in this table of values is 2n:
- n is the term number
- 2 is the multiplier.
So, if n, the term number, is 3, then the term value will be 2(3) or 6.
If we were to represent the first three term values using tiles, it might look like this:
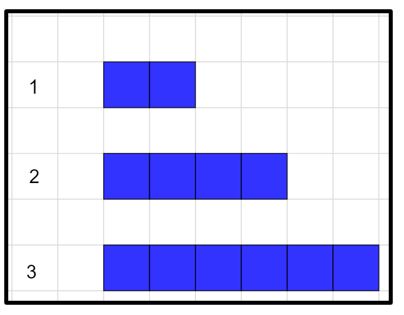
2 – Alter the code so that the table of values outputted represents the expression 3n.
Hint: You will need to alter the multiplier in your code.
The first three term values for this new expression are shown below using tiles:
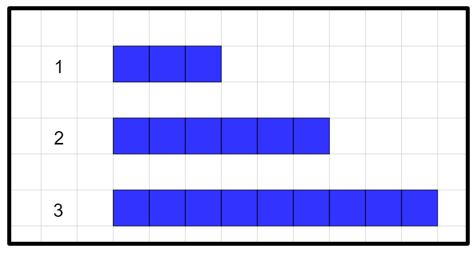
Your output should look like this:
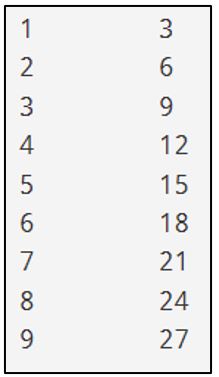
4 – The word “for” indicates the start of a loop that is repeated a certain number of times.
In this case, the termNumber will start at 1 and go up to but not including 10.
So, it will be repeated 9 times.
Alter the code so that it will output numbers 1 to 100, including 100 when executed.
Hint: You will need to change one of the numbers in the brackets for the range.
- 5 – Alter the code to create the output shown.
Record the multiplier and expression required to produce each output.
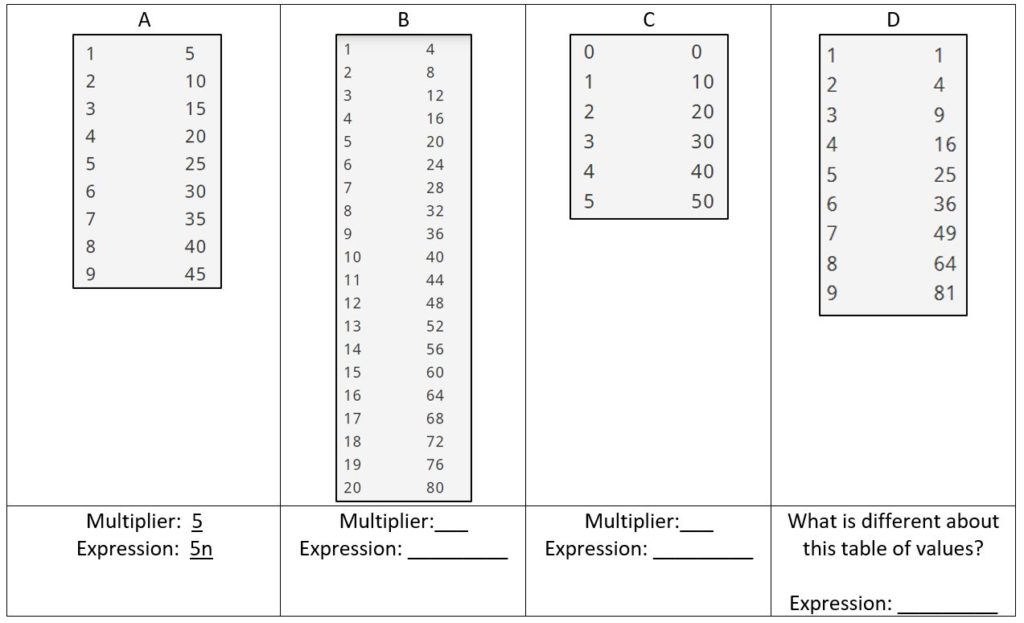
6 – Let’s add a constant to our expressions.
Predict what the output will be when the following code is executed:
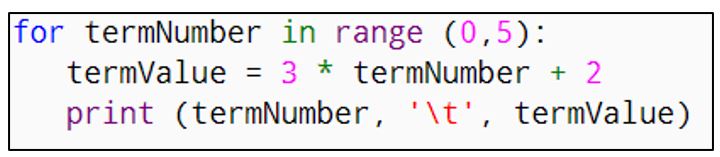
Type the code into the console now to check if you were correct!
In this case, the multiplier is 3 and the constant is 2. The expression is 3n + 2.
7 – Alter the code to create the output shown.
Record the multiplier, constant and expression required to produce each output.
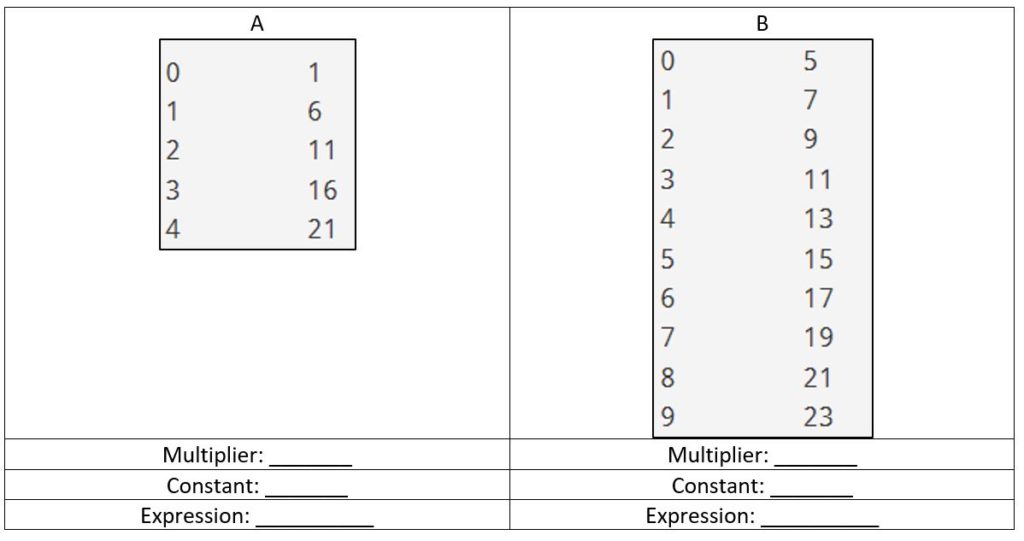
8 – For each of the programs below, record the multiplier, the constant and the expression, then predict the output.
Check to see if you were correct by typing the code and running the programs yourself.
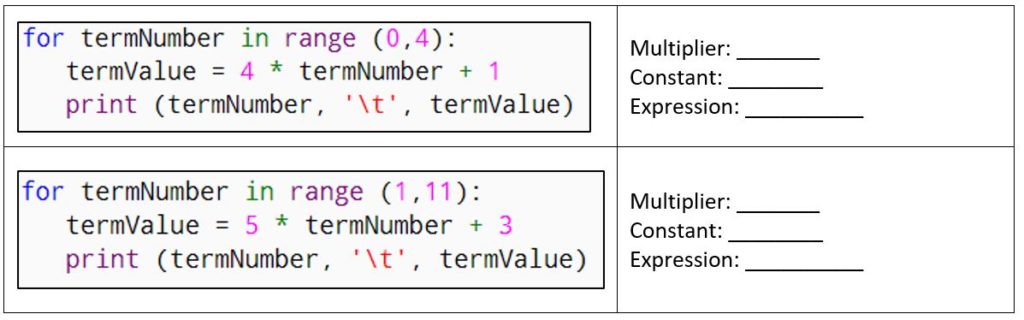
Practice – Representing Linear Expressions through Tables of Values using Code
Part A.
Select any four (or more) of the outputs below and write the code required to create each output. You may choose your four (or more) outputs from any of the Challenging, More Challenging and Super Challenging categories below.
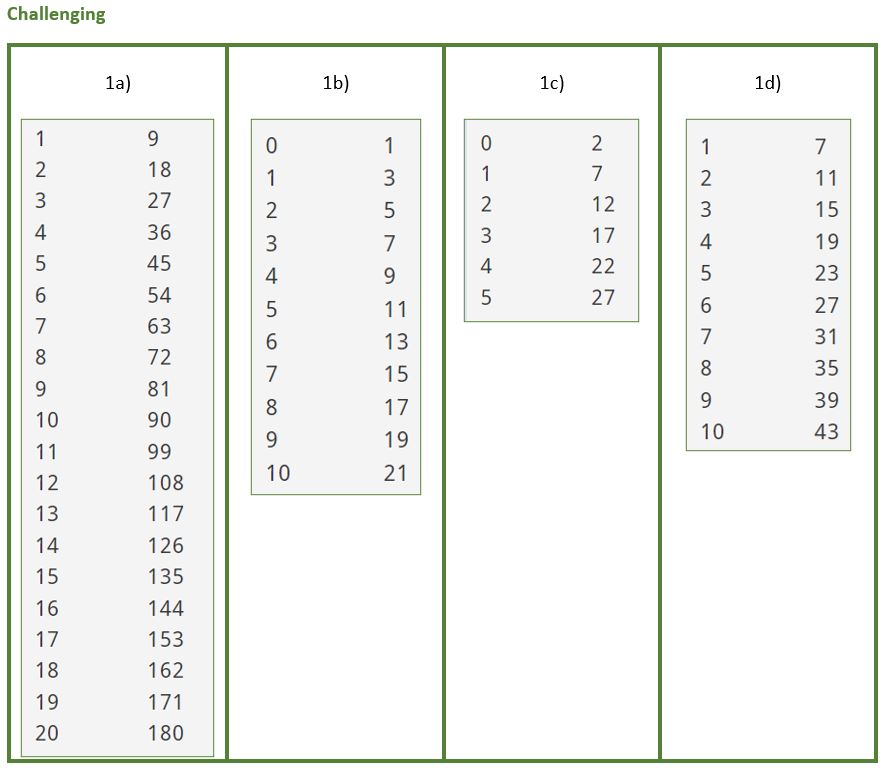
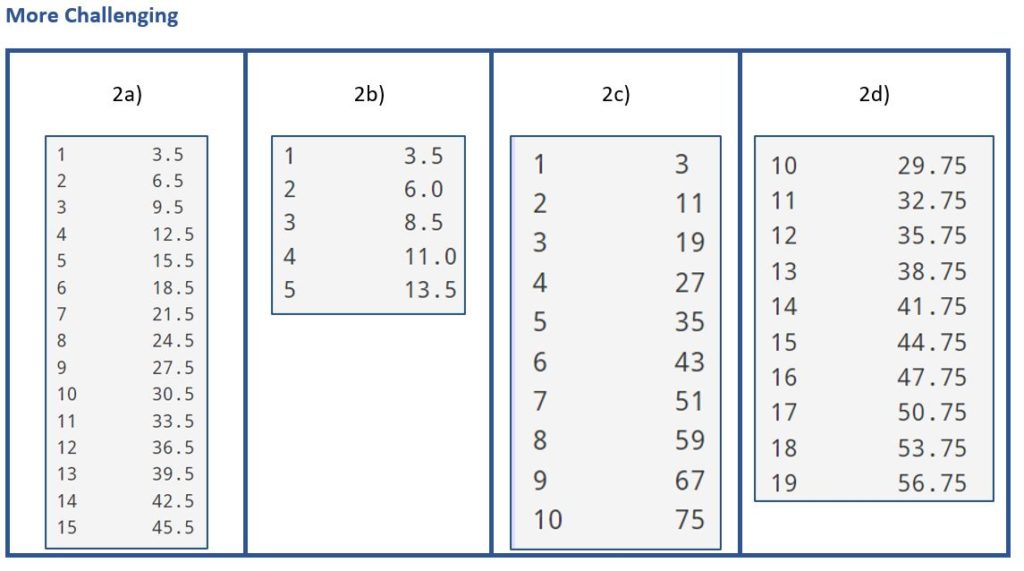
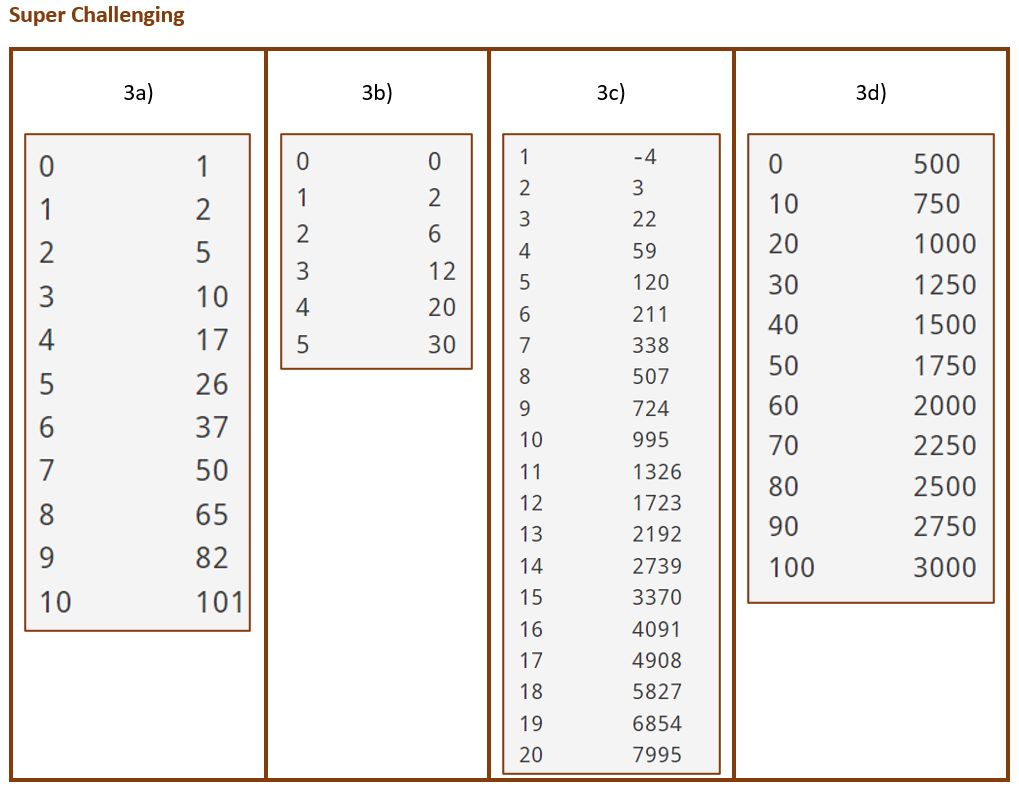
Part B.
Create your own tables of values by writing code to represents the expressions in Python.
See if your classmates can figure out the expressions you have represented in the tables of values!
Prepared by: Lisa Anne Floyd and Steven Floyd